View
state란?
state는 컴포넌트에 대한 데이터 또는 정보를 포함하는 데 쓰이는 리액트 내장 객체다.
- State는 컴포넌트 내에서 지속적으로 변경이 일어나는 값을 관리하기 위해 사용한다.
- State의 값을 변경하기 위해서는 반드시 setState 함수를 이용한다,
- State는 변경되면 자동으로 재렌더링 된다.
useState : 컴포넌트의 상태를 관리하는 함수로 현재의 state 값과 이 값을 업데이트하는 함수를 쌍으로 제공한다.
const [state, setState] = useState(초기값);
- value : 값을 저장하는 변수
- modifier : value 값 변경이 가능하며 자동으로 re-rendering 해준다.
예제)
import React, { useState } from 'react';
function Example() {
// "count"라는 새로운 상태 값을 정의합니다.
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
📌 Hook이란?
더보기
React 에서 기존에 사용하던 Class를 이용한 코드를 작성할 필요 없이 state와 여러 React 기능을 사용할 수 있도록 만든 라이브러리로 React state를 함수 컴포넌트안에서 사용할 수 있게 해준다.
const Example = (props) => {
// 여기서 Hook을 사용할 수 있습니다!
return <div />;
}
function Example(props) {
// 여기서 Hook을 사용할 수 있습니다!
return <div />;
}
🤔 언제 Hook을 사용할까?
함수 컴포넌트를 사용하던 중 state를 추가하고 싶을 때 클래스 컴포넌트로 바꾸곤 했지만 이제 함수 컴포넌트 안에서 Hook을 이용하여 state를 사용할 수 있다.
MinutesToHours Converter 구현
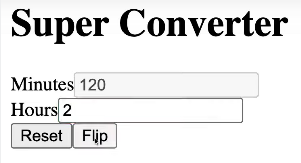
<!DOCTYPE html>
<html>
<body>
<div id="root"></div>
</body>
<script src="https://unpkg.com/react@17.0.2/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@17.0.2/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/@babel/standalone/babel.min.js"></script>
<script type="text/babel">
function App() {
const [amount, setAmount] = React.useState(0);
const [inverted, setInverted] = React.useState(false);
const onChange = (event) => {
setAmount(event.target.value);
};
const reset = () => {
setAmount(0);
};
const onInvert = () => {
reset();
setInverted((current) => !current);
};
return (
<div>
<h1>Super Converter</h1>
<div>
<label htmlFor="minutes">Minutes</label>
<input
value={inverted ? amount * 60 : amount}
id="minutes"
placeholer="Minutes"
type="number"
onChange={onChange}
disabled={inverted === true}
/>
</div>
<div>
<label htmlFor="hours">Hours</label>
<input
value={inverted ? amount : Math.round(amount/60)}
id="hours"
placeholer="Hours"
type="number"
onChange={onChange}
disabled={inverted === false}
/>
</div>
<button onClick={reset}>Reset</button>
<button onClick={onInvert}>{inverted ? "Turn Back" : "Invert"}</button>
</div>
);
}
const root = document.getElementById("root");
ReactDOM.render(<App/>, root);
</script>
</html>
Unit Converter 구현
컴포넌트 분리 (분할 정복)
<!DOCTYPE html>
<html>
<body>
<div id="root"></div>
</body>
<script src="https://unpkg.com/react@17.0.2/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@17.0.2/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/@babel/standalone/babel.min.js"></script>
<script type="text/babel">
function MinutesToHours() {
const [amount, setAmount] = React.useState(0);
const [inverted, setInverted] = React.useState(false);
const onChange = (event) => {
setAmount(event.target.value);
};
const reset = () => {
setAmount(0);
};
const onInvert = () => {
reset();
setInverted((current) => !current);
};
return (
<div>
<div>
<label htmlFor="minutes">Minutes</label>
<input
value={inverted ? amount * 60 : amount}
id="minutes"
placeholer="Minutes"
type="number"
onChange={onChange}
disabled={inverted === true}
/>
</div>
<div>
<label htmlFor="hours">Hours</label>
<input
value={inverted ? amount : Math.round(amount/60)}
id="hours"
placeholer="Hours"
type="number"
onChange={onChange}
disabled={inverted === false}
/>
</div>
<button onClick={reset}>Reset</button>
<button onClick={onInvert}>{inverted ? "Turn Back" : "Invert"}</button>
</div>
);
}
function KmToMiles() {
// KmToMile Code
return (
<div>
KmToMiles
</div>
);
}
function App() {
const [index, setIndex] = React.useState("xx");
const onSelect = (event) => {
setIndex(event.target.value);
};
return (
<div>
<h1>Super Converter</h1>
<select value={index} onChange={onSelect}>
<option value="xx">Select your units</option>
<option value="0">Minutes & Hours</option>
<option value="1">Km & Miles</option>
</select>
<hr/>
{index === "xx" ? "Please select your units" : null}
{index === "0" ? <MinutesToHours/> : null}
{index === "1" ? <KmToMiles/> : null}
</div>
);
}
const root = document.getElementById("root");
ReactDOM.render(<App/>, root);
</script>
</html>
단위 변환기 전체코드
<!DOCTYPE html>
<html>
<body>
<div id="root"></div>
</body>
<script src="https://unpkg.com/react@17.0.2/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@17.0.2/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/@babel/standalone/babel.min.js"></script>
<script type="text/babel">
function MinutesToHours() {
const [amount, setAmount] = React.useState(0);
const [inverted, setInverted] = React.useState(false);
const onChange = (event) => {
setAmount(event.target.value);
};
const reset = () => {
setAmount(0);
};
const onInvert = () => {
reset();
setInverted((current) => !current);
};
return (
<div>
<div>
<label htmlFor="minutes">Minutes</label>
<input
value={inverted ? amount * 60 : amount}
id="minutes"
placeholer="Minutes"
type="number"
onChange={onChange}
disabled={inverted === true}
/>
</div>
<div>
<label htmlFor="hours">Hours</label>
<input
value={inverted ? amount : Math.round(amount/60)}
id="hours"
placeholer="Hours"
type="number"
onChange={onChange}
disabled={inverted === false}
/>
</div>
<button onClick={reset}>Reset</button>
<button onClick={onInvert}>{inverted ? "Turn Back" : "Invert"}</button>
</div>
);
}
function KmToMiles() {
const [amount, setAmount] = React.useState(0);
const [inverted, setInverted] = React.useState(false);
const onChange = (event) => {
setAmount(event.target.value);
};
const reset = () => {
setAmount(0);
};
const onInvert = () => {
reset();
setInverted((current) => !current);
};
return (
<div>
<div>
<label htmlFor="km">Km</label>
<input
value={inverted ? amount * 1.609 : amount}
id="km"
placeholer="Km"
type="number"
onChange={onChange}
disabled={inverted === true}
/>
</div>
<div>
<label htmlFor="miles">Miles</label>
<input
value={inverted ? amount : amount/1.609}
id="miles"
placeholer="Miles"
type="number"
onChange={onChange}
disabled={inverted === false}
/>
</div>
<button onClick={reset}>Reset</button>
<button onClick={onInvert}>{inverted ? "Turn Back" : "Invert"}</button>
</div>
);
}
function App() {
const [index, setIndex] = React.useState("xx");
const onSelect = (event) => {
setIndex(event.target.value);
};
return (
<div>
<h1>Super Converter</h1>
<select value={index} onChange={onSelect}>
<option value="xx">Select your units</option>
<option value="0">Minutes & Hours</option>
<option value="1">Km & Miles</option>
</select>
<hr/>
{index === "xx" ? "Please select your units" : null}
{index === "0" ? <MinutesToHours/> : null}
{index === "1" ? <KmToMiles/> : null}
</div>
);
}
const root = document.getElementById("root");
ReactDOM.render(<App/>, root);
</script>
</html>
728x90
'Frontend > React' 카테고리의 다른 글
[React] #5 Effects- ReactJS 로 영화 웹 서비스 만들기 (0) | 2023.01.19 |
---|---|
[React] #4 Props - ReactJS 로 영화 웹 서비스 만들기 (0) | 2023.01.19 |
[React] React.js란? (0) | 2022.12.13 |
reply